Functional Programming In C
Functional programming is a programming paradigm in C# that is frequently combined with object oriented programming. C# enables you to use imperative programming using object-oriented concepts, but you can also use declarative programming.
Many functional programming articles teach abstract functional techniques. That is, composition, pipelining, higher order functions. This one is different. It shows examples of imperative, unfunctional code that people write every day and translates these examples to a functional style.The first section of the article takes short, data transforming loops and translates them into functional maps and reduces.
Libro de paulo coelho online. [Read] Free Download PDF Brida Paulo Coelho Books. Brida Paulo Coelho. Brida Paulo Coelho PDF. Brida Paulo Coelho PDF Books this is the book you are looking for, from the many other titles of. Brida Paulo Coelho PDF books, here is alsoavailable other sources of this Brida Paulo Coelho. It is the story of a beautiful young Irish girl and her quest for knowledge. On her journey she meets a wise man who teaches her to overcome fear and a woman who teaches her how to dance to the hidden music of the world. They see in her a gift, but must let her make her own voyage of discovery. As Brida seeks her. Free aleph paulo coelho epub paulo coelho free ebook aleph paulo coelho ebook free english. Free aleph ebook free the alchemist by paulo coelho ebook free pdf brida paulo coelho ebook. Free paulo coelho o aleph free paulo coelho alchemist pdf aleph paulo coelho pdf english. Free paulo coelho download free ebook. This is the story of Brida, a young Irish girl, and her quest for knowledge. She has long been interested in various aspects of magic but is searching. Jul 28, 2013. Dear Internet Archive Supporter. I ask only once a year: please help the Internet Archive today. We're an independent, non-profit website that the entire world depends on. Most can't afford to donate, but we hope you can. The average donation is about $41. If everyone chips in $5, we can keep this going.
The second section takes longer loops, breaks them up into units and makes each unit functional. The third section takes a loop that is a long series of successive data transformations and decomposes it into a functional pipeline.The examples are in Python, because many people find Python easy to read. A number of the examples eschew pythonicity in order to demonstrate functional techniques common to many languages: map, reduce, pipeline. All of the examples are in Python 2. A guide ropeWhen people talk about functional programming, they mention a dizzying number of “functional” characteristics. They mention immutable data 1, first class functions 2 and tail call optimisation 3.
These are language features that aid functional programming. They mention mapping, reducing, pipelining, recursing, currying 4 and the use of higher order functions. These are programming techniques used to write functional code. They mention parallelization 5, lazy evaluation 6 and determinism 7.
Programming In C Kochan
These are advantageous properties of functional programs.Ignore all that. Functional code is characterised by one thing: the absence of side effects. It doesn’t rely on data outside the current function, and it doesn’t change data that exists outside the current function. Every other “functional” thing can be derived from this property.
Use it as a guide rope as you learn.This is an unfunctional function. Sum = reduce ( lambda a, x: a + x0, 1, 2, 3, 4 ) print sum # = 10x is the current item being iterated over. A is the accumulator. It is the value returned by the execution of the lambda on the previous item.
Reduce walks through the items. For each one, it runs the lambda on the current a and x and returns the result as the a of the next iteration.What is a in the first iteration? There is no previous iteration result for it to pass along. Reduce uses the first item in the collection for a in the first iteration and starts iterating at the second item. That is, the first x is the second item.This code counts how often the word 'Sam' appears in a list of strings. Sentences = 'Mary read a story to Sam and Isla.'
, 'Isla cuddled Sam.' , 'Sam chortled.'
samcount = reduce ( lambda a, x: a + x. Count ( 'Sam' ), sentences, 0 )How does this code come up with its initial a? The starting point for the number of incidences of 'Sam' cannot be 'Mary read a story to Sam and Isla.' The initial accumulator is specified with the third argument to reduce. This allows the use of a value of a different type from the items in the collection.Why are map and reduce better?First, they are often one-liners.Second, the important parts of the iteration - the collection, the operation and the return value - are always in the same places in every map and reduce.Third, the code in a loop may affect variables defined before it or code that runs after it. By convention, maps and reduces are functional.Fourth, map and reduce are elemental operations.
Every time a person reads a for loop, they have to work through the logic line by line. There are few structural regularities they can use to create a scaffolding on which to hang their understanding of the code. In contrast, map and reduce are at once building blocks that can be combined into complex algorithms, and elements that the code reader can instantly understand and abstract in their mind. “Ah, this code is transforming each item in this collection. It’s throwing some of the transformations away. It’s combining the remainder into a single output.”Fifth, map and reduce have many friends that provide useful, tweaked versions of their basic behaviour. For example: filter, all, any and find.Exercise 2.
Try rewriting the code below using map, reduce and filter. Filter takes a function and a collection.
It returns a collection of every item for which the function returned True. From random import random def movecars : for iin enumerate ( carpositions ): if random 0.3: carpositions i += 1 def drawcar ( carposition ): print '-'.
carposition def runstepofrace : global time time -= 1 movecars def draw : print ' for carposition in carpositions: drawcar ( carposition ) time = 5 carpositions = 1, 1, 1 while time: runstepofrace draw To understand this program, the reader just reads the main loop. “If there is time left, run a step of the race and draw. Check the time again.” If the reader wants to understand more about what it means to run a step of the race, or draw, they can read the code in those functions.There are no comments any more. The code describes itself.Splitting code into functions is a great, low brain power way to make code more readable.This technique uses functions, but it uses them as sub-routines.
They parcel up code. The code is not functional in the sense of the guide rope. The functions in the code use state that was not passed as arguments.
They affect the code around them by changing external variables, rather than by returning values. To check what a function really does, the reader must read each line carefully. If they find an external variable, they must find its origin. They must see what other functions change that variable. Remove stateThis is a functional version of the car race code. Def zero ( s ): if s 0 '0': return s 1: def one ( s ): if s 0 '1': return s 1:zero takes a string, s.
If the first character is '0', it returns the rest of the string. If it is not, it returns None, the default return value of Python functions.
One does the same, but for a first character of '1'.Imagine a function called rulesequence. It takes a string and a list of rule functions of the form of zero and one. It calls the first rule on the string.
Unless None is returned, it takes the return value and calls the second rule on it. Unless None is returned, it takes the return value and calls the third rule on it. And so forth.
If any rule returns None, rulesequence stops and returns None. Otherwise, it returns the return value of the final rule.This is some sample input and output. Def rulesequence ( s, rules ): if s None or not rules: return s else: return rulesequence ( rules 0 ( s ), rules 1:) Use pipelinesIn the previous section, some imperative loops were rewritten as recursions that called out to auxiliary functions.
In this section, a different type of imperative loop will be rewritten using a technique called a pipeline.The loop below performs transformations on dictionaries that hold the name, incorrect country of origin and active status of some bands. Print pipelineeach ( bandssetcanadaascountry, strippunctuationfromname, capitalizenames )This code is easy to understand.
It gives the impression that the auxiliary functions are functional because they seem to be chained together. The output from the previous one comprises the input to the next. If they are functional, they are easy to verify.
They are also easy to reuse, easy to test and easy to parallelize.The job of pipelineeach is to pass the bands, one at a time, to a transformation function, like setcanadaascountry. After the function has been applied to all the bands, pipelineeach bundles up the transformed bands.
Then, it passes each one to the next function.Let’s look at the transformation functions. Def assoc ( d, key, value ): from copy import deepcopy d = deepcopy ( d ) d key = value return d def setcanadaascountry ( band ): return assoc ( band, 'country', 'Canada' ) def strippunctuationfromname ( band ): return assoc ( band, 'name', band 'name'. Replace ( '.' , ' )) def capitalizenames ( band ): return assoc ( band, 'name', band 'name'. Title )Each one associates a key on a band with a new value. There is no easy way to do this without mutating the original band.
Functional Programming In C++11
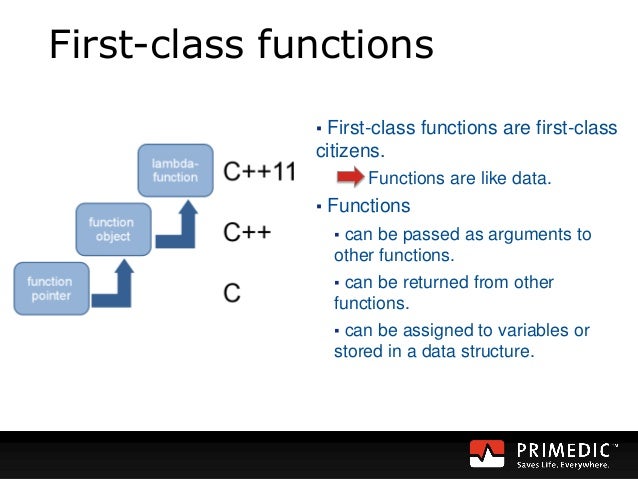
Assoc solves this problem by using deepcopy to produce a copy of the passed dictionary. Each transformation function makes its modification to the copy and returns that copy.Everything seems fine.
Band dictionary originals are protected from mutation when a key is associated with a new value. But there are two other potential mutations in the code above. In strippunctuationfromname, the unpunctuated name is generated by calling replace on the original name.
In capitalizenames, the capitalized name is generated by calling title on the original name. If replace and title are not functional, strippunctuationfromname and capitalizenames are not functional.Fortunately, replace and title do not mutate the strings they operate on. This is because strings are immutable in Python. When, for example, replace operates on a band name string, the original band name is copied and replace is called on the copy. Phew.This contrast between the mutability of strings and dictionaries in Python illustrates the appeal of languages like Clojure. The programmer need never think about whether they are mutating data. They aren’t.Exercise 4.
Try and write the pipelineeach function. Think about the order of operations. The bands in the array are passed, one band at a time, to the first transformation function. The bands in the resulting array are passed, one band at a time, to the second transformation function.
And so forth.My solution. Def assoc ( d, key, value ): from copy import deepcopy d = deepcopy ( d ) d key = value return d def call ( fn, key ): def applyfn ( record ): return assoc ( record, key, fn ( record.
Get ( key ))) return applyfnThere is a lot going on here. Let’s take it piece by piece.One. Call is a higher order function. A higher order function takes a function as an argument, or returns a function. Or, like call, it does both.Two. Applyfn looks very similar to the three transformation functions.
It takes a record (a band). It looks up the value at recordkey. It calls fn on that value.
It assigns the result back to a copy of the record. It returns the copy.Three.
Call does not do any actual work. Applyfn, when called, will do the work. In the example of using pipelineeach above, one instance of applyfn will set 'country' to 'Canada' on a passed band. Another instance will capitalize the name of a passed band.Four. When an applyfn instance is run, fn and key will not be in scope.
They are neither arguments to applyfn, nor locals inside it. But they will still be accessible. When a function is defined, it saves references to the variables it closes over: those that were defined in a scope outside the function and that are used inside the function. When the function is run and its code references a variable, Python looks up the variable in the locals and in the arguments. If it doesn’t find it there, it looks in the saved references to closed over variables. This is where it will find fn and key.Five.
There is no mention of bands in the call code. That is because call could be used to generate pipeline functions for any program, regardless of topic. Functional programming is partly about building up a library of generic, reusable, composable functions.Good job. Closures, higher order functions and variable scope all covered in the space of a few paragraphs. Have a nice glass of lemonade.There is one more piece of band processing to do. That is to remove everything but the name and country.
Extractnameandcountry can pull that information out.
It’s possible that the post title above left you scratching your head. “Functional programming in C#. Is that even a thing?” Well, it’s definitely a thing. Allow me to clarify.Most people would classify C# as an object-oriented language, but it’s possible that you, as a.NET/C# developer, have been using functional programming concepts without even knowing it.Make no mistake. Using functional-programming-inspired features is not the same as programming in a pure functional language, like Haskell. Or even a hybrid language but with stronger emphasis on the functional side, like F#.However, employing functional programming concepts can improve the. And that’s what today’s post is about.
Functional Programming In C# 7
I’ll just first briefly cover the attractions of functional programming and why it makes sense to apply it even when using a so-called object-oriented language. Then I’ll show you how you’ve already been using some functional style in your C# code, even if you’re not aware of it. I’ll tell you how you can apply functional thinking to your code in order to make it cleaner, safer, and more expressive.
Thanks for the article. I’ve recently been trying to imagine what “functional” versions of my code would look like. Then I realized that the LINQ I’ve been writing for years illustrates the concepts.What I appreciate most is explaining Map, Filter, and Reduce. These are things I already do but without the terminology.
Putting words with concepts is a big deal. It gives me more internal language to describe my thoughts and helps me relate them to other things I read. You wouldn’t think a few words would make that much difference, but they do. It’s so much easier to grasp new concepts when your mind has the words to think about them.